Back to all posts
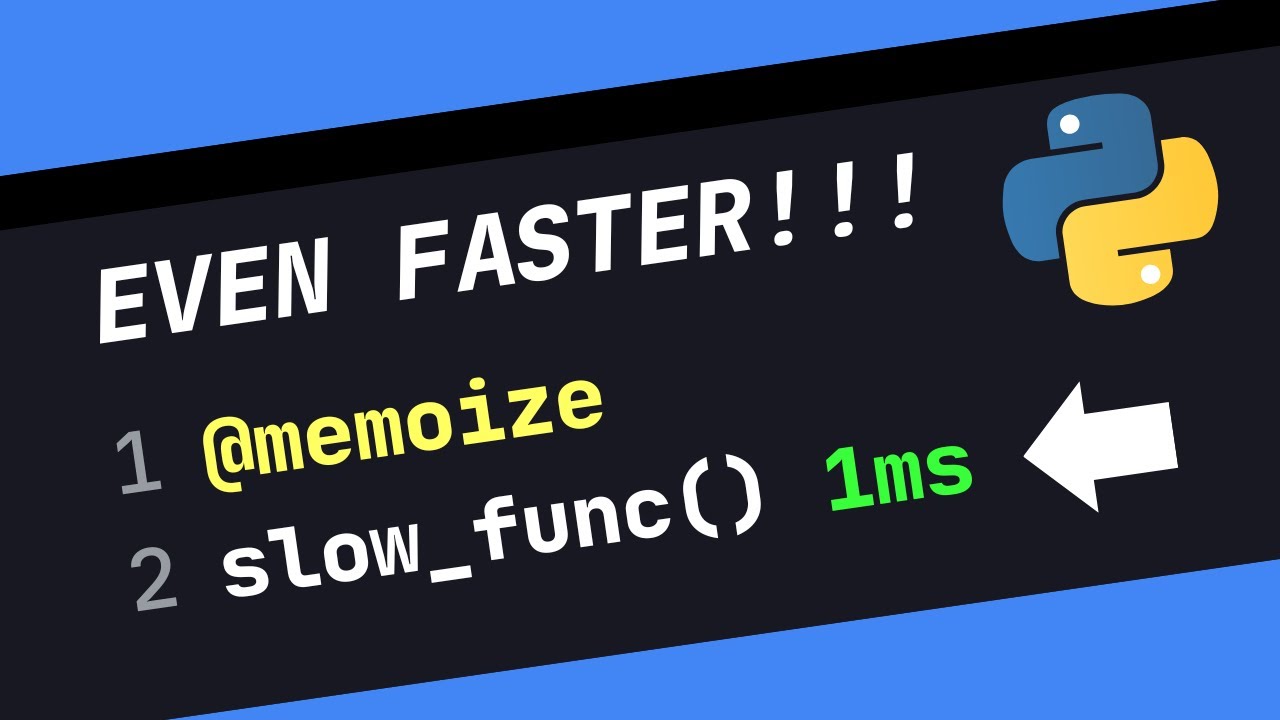
January 22, 2024•22 min read•By Aisha Iftikhar
Python Performance Optimization Techniques
Python Performance Optimization: Unleashing the Full Potential of Your Applications
Python's elegance and readability are unparalleled, but performance can sometimes be a concern. This guide explores advanced techniques to supercharge your Python applications.
Profiling: The Diagnostic Approach
Utilize tools like cProfile and line_profiler to identify and address performance bottlenecks.
import cProfile
import pstats
def complex_calculation():
# Your computational logic here
return [x**2 for x in range(100000)]
# Profiling the function
profiler = cProfile.Profile()
profiler.enable()
result = complex_calculation()
profiler.disable()
# Print performance statistics
stats = pstats.Stats(profiler).sort_stats('cumulative')
stats.print_stats()
Leveraging Built-in Optimizations
Use NumPy and Pandas for high-performance numerical computations and data manipulation.
import numpy as np
# Numba JIT compilation for speed
from numba import jit
@jit(nopython=True)
def fast_computation(x):
result = 0
for i in range(len(x)):
result += x[i] ** 2
return result
# Benchmark
data = np.random.rand(1000000)
result = fast_computation(data)
Concurrency and Parallelism
Implement asyncio and multiprocessing to handle I/O-bound and CPU-bound tasks efficiently.
import asyncio
import aiohttp
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
urls = ['http://example.com', 'http://another.com']
tasks = [fetch_url(url) for url in urls]
results = await asyncio.gather(*tasks)
Conclusion
Performance optimization is an art. The goal is not just speed, but creating efficient, maintainable, and elegant code.
Optimize wisely, measure consistently, and never compromise on code quality!